目录Swing 常用控件1. JFrame2. JLabel3. JButton4. JTextArea5. JTextField6. JPasswordField7. JRadioButton8. JCheckBox9. JComboBox10. JList
Swing 常用控件
Swing 常用控件包括 JFrame、JLabel、JButton、JTextArea 等;
1. JFrame
在 Swing 组件中,最常见的一个控件就是 JFrame,它和 Frame 一样是一个独立存在的顶级窗口,不能放置在其他容器中。JFrame 支持通用窗口所有的基本功能,例如窗口最小化、设定窗口大小等
使用 JFrame 生成窗体:
import javax.swing.*;
import java.awt.*;
public class Test extends JFrame {
public Test() {
this.setTitle("Regino");
this.setSize(300, 200);
// 定义一个按钮组件
JButton bt = new JButton("Click Me");
// 设置流式布局管理器
this.setLayout(new FlowLayout());
// 添加按钮组件
this.add(bt);
// 设置单击关闭按钮时的默认操作
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
this.setVisible(true);
}
public static void main(String[] args) {
new Test();
}
}
效果图:
JFrame 和 Frame 窗体的效果基本相同,但是 JFrame 类提供了关闭窗口的功能:在程序中不需要添加窗体监听器,只需要调用 setDefaultCloseOperation() 方法,然后将常量 JFrame.EXIT_ON_CLOSE 作为参数传入即可;
2. JLabel
JLabel 组件用来显示文本和图像,可以只显示其中之一,也可以两者同时显示。JLabel 类提供了一系列用来设置标签的方法,例如通过 setText(String text) 方法设置标签显示的文本,通过 setFont(Font font) 方法设置标签文本的字体及大小,通过 setHorizontalAlignment(int alignment),方法设置文本的显示位置,该方法的参数可以从 JLabel 类提供的与水平布置方式有关的静态常量中选择;
静态常量 |
常量值 |
标签内容显示位置 |
LEFT |
2 |
靠左侧显示 |
CENTER |
0 |
居中显示 |
RIGHT |
4 |
靠右侧显示 |
如果需要在标签中显示图片,可以通过 setIcon(Icon icon) 方法设置,如果想在标签中既显示文本又显示图片,可以通过 setHorizontalTextPosition(int textPosition) 方法设置文字相对图片在水平方向额显示位置,还可以通过 setVerticalTextPosition(int textPosition) 方法设置文字相对图片在垂直方向的显示位置,该方法的入口参数可以从 JLabel 类提供的与垂直布置方式有关的静态常量中选择;
静态常量 |
常量值 |
标签内容显示位置 |
TOP |
1 |
文字显示在图片的上方 |
CENTER |
0 |
文字与图片在垂直方向重叠显示 |
Bottom |
3 |
文字显示在图片的下方 |
同时显示文本和图片标签:
import javax.swing.*;
import java.awt.*;
public class Test extends JFrame { // 继承窗体类JFrame
public static void main(String args[]) {
Test frame = new Test();
frame.setVisible(true); // 设置窗体可见,默认为不可见
}
public Test() {
super(); // 继承父类的构造方法
setTitle("Regino"); // 设置窗体的标题
setBounds(100, 100, 500, 375); // 设置窗体的显示位置及大小
getContentPane().setLayout(null); // 设置为不采用任何布局管理器
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);// 设置窗体关闭按钮的动作为退出
final JLabel label = new JLabel(); // 创建标签对象
label.setBounds(0, 0, 492, 341); // 设置标签的显示位置及大小
label.setText("Icon is Here"); // 设置标签显示文字
label.setFont(new Font("", Font.BOLD, 22)); // 设置文字的字体及大小
label.setHorizontalAlignment(JLabel.CENTER); // 设置标签内容居中显示
label.setIcon(new ImageIcon("C:\\Users\\80626\\Desktop\\pre.png")); // 设置标签显示图片
label.setHorizontalTextPosition(JLabel.CENTER); // 设置文字相对图片在水平方向的显示位置
label.setVerticalTextPosition(JLabel.BOTTOM); // 设置文字相对图片在垂直方向的显示位置
getContentPane().add(label); // 将标签添加到窗体中
}
}
效果图:
创建图片对象的时候,如果地址仅为图片名称,则需要将图片和相应的类文件放在同一路径下;
3. JButton
JButton 组件是最简单的按钮组件,只是在按下和释放两个状态之间切换,可以通过捕获按下并释放的动作执行一些操作,从而完成和用户的交互。JButton 类提供了一系列用来设置按钮的方法,例如通过 setText(String text) 方法设置按钮的标签文本;
实现一个典型的按钮;
import javax.swing.*;
import java.awt.*;
public class Test extends JFrame { // 继承窗体类JFrame
public static void main(String args[]) {
Test frame = new Test();
frame.setVisible(true); // 设置窗体可见,默认为不可见
}
public Test() {
super(); // 继承父类的构造方法
setTitle("Regino"); // 设置窗体的标题
setBounds(100, 100, 520, 395); // 设置窗体的显示位置及大小
getContentPane().setLayout(null); // 设置为不采用任何布局管理器
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);// 设置窗体关闭按钮的动作为退出
final JButton button = new JButton(); // 创建按钮对象
button.setMargin(new Insets(0, 0, 0, 0)); // 设置按钮边框和标签之间的间隔
button.setContentAreaFilled(false); // 设置不绘制按钮的内容区域
button.setBorderPainted(false); // 设置不绘制按钮的边框
button.setIcon(new ImageIcon("C:\\Users\\80626\\Desktop\\land.jpg")); // 设置默认情况下按钮显示的图片
button.setRolloverIcon(new ImageIcon("C:\\Users\\80626\\Desktop\\land_over.png")); // 设置光标经过时显示的图片
button.setPressedIcon(new ImageIcon("C:\\Users\\80626\\Desktop\\land_pressed.jpg"));// 设置按钮被按下时显示的图片
button.setBounds(10, 10, 480, 355); // 设置标签的显示位置及大小
getContentPane().add(button); // 将按钮添加到窗体中
}
}
效果图:
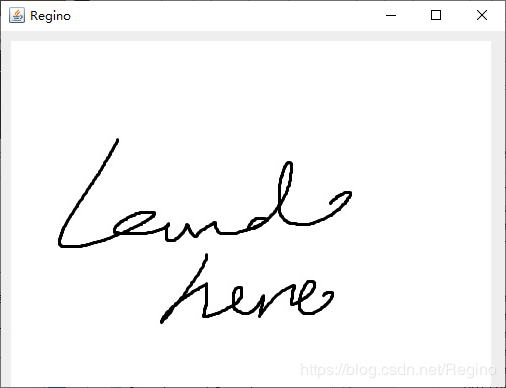
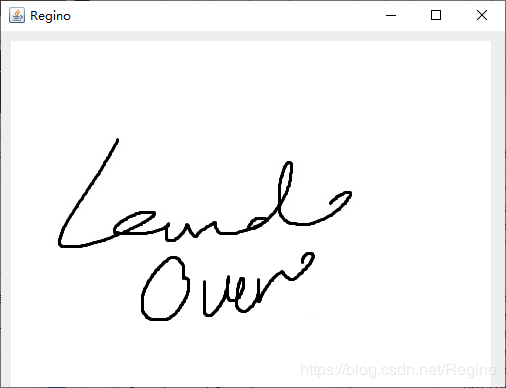
4. JTextArea
JTextArea 组件实现一个文本域,文本域可以接收用户输入的多行文本。在创建文本域时,可以通过 setLineWrap(boolean wrap) 方法设置文本是否自动换行,默认为 false,即不自动换行,否则为自动换行;
常用方法:
方法 |
说明 |
append(String str) |
将指定文本追加到文档结尾 |
insert(String str, int pos) |
将指定文本插入指定位置 |
replaceRange(String str, int start, int end) |
用指定新文本替换从指定的起始位置到结尾位置的文本 |
getColumnWidth() |
获取列的宽度 |
getColumns() |
返回文本域的列数 |
getLineCount() |
确定文本域中实际文本的行数 |
getPreferredSize() |
返回文本域的首选大小 |
getRows() |
返回文本域的行数 |
setLineWrap(boolean wrap) |
设置文本域是否自动换行,默认为 false,即不自动换行 |
实现文本域,并自动换行:
import javax.swing.*;
import java.awt.*;
public class Test extends JFrame { // 继承窗体类JFrame
public static void main(String args[]) {
Test frame = new Test();
frame.setVisible(true); // 设置窗体可见,默认为不可见
}
public Test() {
super(); // 继承父类的构造方法
setTitle("Regino"); // 设置窗体的标题
setBounds(100, 100, 500, 375); // 设置窗体的显示位置及大小
getContentPane().setLayout(null); // 设置为不采用任何布局管理器
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);// 设置窗体关闭按钮的动作为退出
final JLabel label = new JLabel();
label.setText("Note:");
label.setBounds(10, 10, 46, 15);
getContentPane().add(label);
JTextArea textArea = new JTextArea(); // 创建文本域对象
textArea.setColumns(15); // 设置文本域显示文字的列数
textArea.setRows(3); // 设置文本域显示文字的行数
textArea.setLineWrap(true); // 设置文本域自动换行
final JScrollPane scrollPane = new JScrollPane(); // 创建滚动面板对象
scrollPane.setViewportView(textArea); // 将文本域添加到滚动面板中
Dimension dime = textArea.getPreferredSize(); // 获得文本域的首选大小
scrollPane.setBounds(62, 5, dime.width, dime.height); // 设置滚动面板的位置及大小
getContentPane().add(scrollPane); // 将滚动面板添加到窗体中
}
}
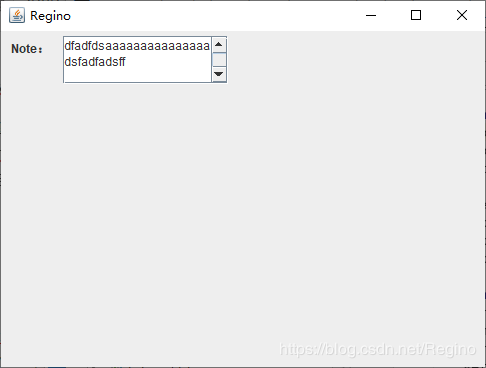
5. JTextField
JTextField 组件实现一个文本框,用来接收用户输入的单行文本信息。如果需要为文本框设置默认文本,可以通过构造函数 JTextField(String text) 创建文本框对象, 例如:JTextField textField= new JTextField (“请输入姓名”); 也可以通过方法 setText(String t) 为文本框设置文本信息,例如:JTextField textField= new JTextField (“请输入姓名”);textField.setText(“请输入姓名”);在设置文本框时,可以通过 setHorizontalAlignment(int alignment) 方法设置文本框内容的水平对齐方式,该方法的入口参数可以从 JTextField 类中的静态常量中选择;
创建文本框:
import javax.swing.*;
import java.awt.*;
public class Test extends JFrame { // 继承窗体类JFrame
public static void main(String args[]) {
Test frame = new Test();
frame.setVisible(true); // 设置窗体可见,默认为不可见
}
public Test() {
super(); // 继承父类的构造方法
setTitle("Regino"); // 设置窗体的标题
setBounds(100, 100, 500, 375); // 设置窗体的显示位置及大小
getContentPane().setLayout(null); // 设置为不采用任何布局管理器
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);// 设置窗体关闭按钮的动作为退出
final JLabel label = new JLabel(); // 创建标签对象
label.setText("Name:"); // 设置标签文本
label.setBounds(10, 10, 46, 15); // 设置标签的显示位置及大小
getContentPane().add(label); // 将标签添加到窗体中
JTextField textField = new JTextField(); // 创建文本框对象
textField.setHorizontalAlignment(JTextField.CENTER); // 设置文本框内容的水平对齐方式
textField.setFont(new Font("", Font.BOLD, 12)); // 设置文本框内容的字体样式
textField.setBounds(62, 7, 120, 21); // 设置文本框的显示位置及大小
getContentPane().add(textField); // 将文本框添加到窗体中
}
}
效果图:
在窗体中实现文本框:
import javax.swing.*;
import java.awt.*;
public class Test extends JFrame { // 继承窗体类JFrame
JTextField nameTextField, addressTextField ;
Container message;
JLabel nameLabel,addresLlabel;
JButton findButton;
public static void main(String args[]) {
Test frame = new Test();
frame.setSize(400, 150);
frame.setVisible(true); // 设置窗体可见,默认为不可见
}
public Test() {
super(); // 继承父类的构造方法
message=getContentPane();
message.setLayout(null);
JLabel nameLabel = new JLabel("Name");
nameLabel.setBounds(10, 34, 54, 15);
message.add(nameLabel);
nameTextField = new JTextField();
nameTextField.setBounds(62, 31, 97, 25);
message.add(nameTextField);
nameTextField.setColumns(10);
addresLlabel = new JLabel("Address");
addresLlabel.setBounds(169, 34, 38, 15);
message.add(addresLlabel);
addressTextField = new JTextField();
addressTextField.setBounds(204, 31, 119, 25);
message.add(addressTextField);
addressTextField.setColumns(10);
}
}
效果图:
6. JPasswordField
JPasswordField 组件实现一个密码框,用来接收用户输入的单行文本信息,在密码框中并不显示用户输入的真实信息,而是显示一个指定的回显字符作为占位符。新创建密码框的默认回显字符为*,可以通过 setEchoChar(char c)方法修改回显字符,例如将回显字符修改为#;
创建密码框:
import javax.swing.*;
public class Test extends JFrame { // 继承窗体类JFrame
public static void main(String args[]) {
Test frame = new Test();
frame.setVisible(true); // 设置窗体可见,默认为不可见
}
public Test() {
super(); // 继承父类的构造方法
setTitle("Regino"); // 设置窗体的标题
setBounds(100, 100, 500, 375); // 设置窗体的显示位置及大小
getContentPane().setLayout(null); // 设置为不采用任何布局管理器
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);// 设置窗体关闭按钮的动作为退出
final JLabel label = new JLabel(); // 创建标签对象
label.setText("密码:"); // 设置标签文本
label.setBounds(10, 10, 46, 15); // 设置标签的显示位置及大小
getContentPane().add(label); // 将标签添加到窗体中
JPasswordField passwordField = new JPasswordField(); // 创建密码框对象
passwordField.setEchoChar('*'); // 设置回显字符为‘*’
passwordField.setBounds(62, 7, 150, 21); // 设置密码框的显示位置及大小
getContentPane().add(passwordField); // 将密码框添加到窗体中
}
}
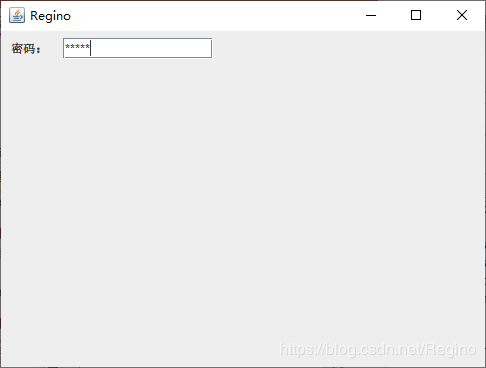
7. JRadioButton
JRadioButton 组件实现一个单选按钮,用户可以很方便地查看单选按钮的状态。JRadioButton 类可以单独使用,也可以与 ButtonGroup 类联合使用。当单独使用时,该单选按钮可以被选定和取消选定;当与ButtonGroup 类联合使用时,则组成了一个单选按钮组,此时用户只能选定按钮组中的一个单选按钮,取消选定的操作将由 ButtonGroup 类自动完成。ButtonGroup 类用来创建一个按钮组,其作用是负责维护该组按钮的开启状态,在按钮组中只能有一个按钮处于开启状态。假设在按钮组中有且仅有 A 按钮处于开启状态,在开启其他按钮时,按钮组将自动关闭 A 按钮的开启状态;
单选按钮:
import javax.swing.*;
public class Test extends JFrame { // 继承窗体类JFrame
public static void main(String args[]) {
Test frame = new Test();
frame.setVisible(true); // 设置窗体可见,默认为不可见
}
public Test() {
super(); // 继承父类的构造方法
setTitle("Regino"); // 设置窗体的标题
setBounds(100, 100, 500, 375); // 设置窗体的显示位置及大小
getContentPane().setLayout(null); // 设置为不采用任何布局管理器
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);// 设置窗体关闭按钮的动作为退出
final JLabel label = new JLabel(); // 创建标签对象
label.setText("Gender:"); // 设置标签文本
label.setBounds(10, 10, 46, 15); // 设置标签的显示位置及大小
getContentPane().add(label); // 将标签添加到窗体中
ButtonGroup buttonGroup = new ButtonGroup(); // 创建按钮组对象
final JRadioButton manRadioButton = new JRadioButton(); // 创建单选按钮对象
buttonGroup.add(manRadioButton); // 将单选按钮添加到按钮组中
manRadioButton.setSelected(true); // 设置单选按钮默认为被选中
manRadioButton.setText("M"); // 设置单选按钮的文本
manRadioButton.setBounds(62, 6, 46, 23); // 设置单选按钮的显示位置及大小
getContentPane().add(manRadioButton); // 将单选按钮添加到窗体中
final JRadioButton womanRadioButton = new JRadioButton();
buttonGroup.add(womanRadioButton);
womanRadioButton.setText("F");
womanRadioButton.setBounds(114, 6, 46, 23);
getContentPane().add(womanRadioButton);
}
}
效果图:
8. JCheckBox
JCheckBox 组件实现一个复选框,该复选框可以被选定和取消选定。可以同时选定多个复选框。用户可以很方便地查看复选框的状态。JCheckBox 类提供了一系列用来设置复选框的方法,例如通过 setText(String text)方法设置复选框的标签文本,通过 setSelected(boolean b) 方法设置复选框的状态,默认情况下复选框未被选中, 当设为 true 时表示复选框被选中;
复选框:
import javax.swing.*;
public class Test extends JFrame { // 继承窗体类JFrame
public static void main(String args[]) {
Test frame = new Test();
frame.setVisible(true); // 设置窗体可见,默认为不可见
}
public Test() {
super(); // 继承父类的构造方法
setTitle("Regino"); // 设置窗体的标题
setBounds(100, 100, 500, 375); // 设置窗体的显示位置及大小
getContentPane().setLayout(null); // 设置为不采用任何布局管理器
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);// 设置窗体关闭按钮的动作为退出
final JLabel label = new JLabel(); // 创建标签对象
label.setText("Hobby:"); // 设置标签文本
label.setBounds(10, 10, 46, 15); // 设置标签的显示位置及大小
getContentPane().add(label); // 将标签添加到窗体中
final JCheckBox readingCheckBox = new JCheckBox(); // 创建复选框对象
readingCheckBox.setText("Read"); // 设置复选框的标签文本
readingCheckBox.setBounds(62, 6, 55, 23); // 设置复选框的显示位置及大小
getContentPane().add(readingCheckBox); // 将复选框添加到窗体中
final JCheckBox musicCheckBox = new JCheckBox();
musicCheckBox.setText("music");
musicCheckBox.setBounds(123, 6, 68, 23);
getContentPane().add(musicCheckBox);
final JCheckBox pingpongCheckBox = new JCheckBox();
pingpongCheckBox.setText("sports");
pingpongCheckBox.setBounds(197, 6, 75, 23);
getContentPane().add(pingpongCheckBox);
}
}
效果图:
9. JComboBox
JComboBox 组件实现一个组合框,用户可以从下拉选项列表中选择相应的值,该选项列表框还可以设置为可编辑的,此时用户可以在框中输入相应的值;
选择框:
import javax.swing.*;
public class Test extends JFrame { // 继承窗体类JFrame
public static void main(String args[]) {
Test frame = new Test();
frame.setVisible(true); // 设置窗体可见,默认为不可见
}
public Test() {
super(); // 继承父类的构造方法
setTitle("Regino"); // 设置窗体的标题
setBounds(100, 100, 500, 375); // 设置窗体的显示位置及大小
getContentPane().setLayout(null); // 设置为不采用任何布局管理器
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);// 设置窗体关闭按钮的动作为退出
final JLabel label = new JLabel(); // 创建标签对象
label.setText("Education:"); // 设置标签文本
label.setBounds(10, 10, 46, 15); // 设置标签的显示位置及大小
getContentPane().add(label); // 将标签添加到窗体中
String[] schoolAges = { "undergraduate", "Master", "Doctor" }; // 创建选项数组
JComboBox comboBox = new JComboBox(schoolAges); // 创建选择框对象
comboBox.setEditable(true); // 设置选择框为可编辑
comboBox.setMaximumRowCount(3); // 设置选择框弹出时显示选项的最多行数
comboBox.insertItemAt(" associate-degree", 0); // 在索引为0的位置插入一个选项
comboBox.setSelectedItem("undergraduate"); // 设置索引为0的选项被选中
comboBox.setBounds(62, 7, 104, 21); // 设置选择框的显示位置及大小
getContentPane().add(comboBox); // 将选择框添加到窗体中
}
}
效果图:
10. JList
JList 组件实现一个列表框,列表框与组合框的主要区别是列表框可以多选,而组合框只能单选。在创建列表框时,需要通过构造函数 JList(Object[] list)
直接初始化该列表框包含的选项。例如创建一个用来选择月份的列表框,具体代码如下:
Integer[] months = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12 };
JList list = new JList(months);
由 JList 组件实现的列表框有 3 种选取模式,可以通过 JList 类的 setSelectionMode(int selectionMode) 方法设置,该方法的参数可以从 ListSelectionModel 类中的静态常量中选择。这 3 种选取模式包括一种单选模式和两种多选模式;
列表框:
import javax.swing.*;
public class Test extends JFrame { // 继承窗体类JFrame
public static void main(String args[]) {
Test frame = new Test();
frame.setVisible(true); // 设置窗体可见,默认为不可见
}
public Test() {
super(); // 继承父类的构造方法
setTitle("Regino"); // 设置窗体的标题
setBounds(100, 100, 500, 375); // 设置窗体的显示位置及大小
getContentPane().setLayout(null); // 设置为不采用任何布局管理器
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);// 设置窗体关闭按钮的动作为退出
final JLabel label = new JLabel(); // 创建标签对象
label.setText("Hobby:"); // 设置标签文本
label.setBounds(10, 10, 46, 15); // 设置标签的显示位置及大小
getContentPane().add(label); // 将标签添加到窗体中
String[] likes = { "Coding", "Coding", "Coding", "Coding", "Coding", "Coding", "Coding" };
JList list = new JList(likes); // 创建列表对象
list.setSelectionMode(ListSelectionModel.MULTIPLE_INTERVAL_SELECTION);
list.setFixedCellHeight(20); // 设置选项高度
list.setVisibleRowCount(4); // 设置选项可见个数
JScrollPane scrollPane = new JScrollPane(); // 创建滚动面板对象
scrollPane.setViewportView(list); // 将列表添加到滚动面板中
scrollPane.setBounds(62, 5, 65, 80); // 设置滚动面板的显示位置及大小
getContentPane().add(scrollPane); // 将滚动面板添加到窗体中
}
}
效果图:
注意:JList 类实现的列表并不提供滚动窗口,如果需要将列表中的选项显示在滚动窗口中,则需要将列表框添加到面板中,然后再将滚动面板添加到窗体中;
作者:Regino
swing
JAVA
java swing