我们用Spring Boot来轻轻松松的生成一个二维码,仅需导入google的zxing工具类就行。
目录
1.引入jar包
2.编写工具类
3.编写控制层代码
4.运行并查看效果
二维码又称为QR Code,就不多说了,生活中处处可见,应用场景相当广泛。
今天我们就来看看怎么用Java生成一个二维码。
1.引入jar包pom.xml中添加:
com.google.zxing
core
3.2.0
com.google.zxing
javase
3.2.0
2.编写工具类
QRCodeUtil.java:
三个主要功能:
1.创建和插入logo图片(二维码正中间那个);
2.生成二维码;
**生成二维码可以重载很多方法
** 最主要的区别就是一种是把二维码图片存放在某个目录下,一种通过输出流直接返回到网页上
** 其他都是一些小区别,比如有没有imgPath(logo图片地址)或needCompress(是否压缩)这些参数
3.解析二维码(代码解析);
package com.erweima.Utils;
import com.google.zxing.*;
import com.google.zxing.client.j2se.BufferedImageLuminanceSource;
import com.google.zxing.common.BitMatrix;
import com.google.zxing.common.HybridBinarizer;
import com.google.zxing.qrcode.decoder.ErrorCorrectionLevel;
import javax.imageio.ImageIO;
import java.awt.*;
import java.awt.geom.RoundRectangle2D;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.OutputStream;
import java.util.Hashtable;
/**
* QRCodeUtil 生成二维码工具类
*/
public class QRCodeUtil {
private static final String CHARSET = "utf-8";
private static final String FORMAT_NAME = "JPG";
// 二维码尺寸
private static final int QRCODE_SIZE = 300;
// LOGO宽度
private static final int WIDTH = 60;
// LOGO高度
private static final int HEIGHT = 60;
/**
* 创建logo图片(二维码正中间那个)
* @param content 内容
* @param imgPath LOGO图片地址
* @param needCompress 是否压缩
* @return
* @throws Exception
*/
private static BufferedImage createImage(String content, String imgPath, boolean needCompress) throws Exception {
Hashtable hints = new Hashtable();
hints.put(EncodeHintType.ERROR_CORRECTION, ErrorCorrectionLevel.H);
hints.put(EncodeHintType.CHARACTER_SET, CHARSET);
hints.put(EncodeHintType.MARGIN, 1);
BitMatrix bitMatrix = new MultiFormatWriter().encode(content, BarcodeFormat.QR_CODE, QRCODE_SIZE, QRCODE_SIZE,
hints);
int width = bitMatrix.getWidth();
int height = bitMatrix.getHeight();
BufferedImage image = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);
for (int x = 0; x < width; x++) {
for (int y = 0; y WIDTH) {
width = WIDTH;
}
if (height > HEIGHT) {
height = HEIGHT;
}
Image image = src.getScaledInstance(width, height, Image.SCALE_SMOOTH);
BufferedImage tag = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);
Graphics g = tag.getGraphics();
g.drawImage(image, 0, 0, null); // 绘制缩小后的图
g.dispose();
src = image;
}
// 插入LOGO
Graphics2D graph = source.createGraphics();
int x = (QRCODE_SIZE - width) / 2;
int y = (QRCODE_SIZE - height) / 2;
graph.drawImage(src, x, y, width, height, null);
Shape shape = new RoundRectangle2D.Float(x, y, width, width, 6, 6);
graph.setStroke(new BasicStroke(3f));
graph.draw(shape);
graph.dispose();
}
/**
* 当文件夹不存在时,mkdirs会自动创建多层目录,区别于mkdir.(mkdir如果父目录不存在则会抛出异常)
* @param destPath
*/
public static void mkdirs(String destPath) {
File file = new File(destPath);
if (!file.exists() && !file.isDirectory()) {
file.mkdirs();
}
}
/**
* 生成二维码可以重载很多方法
* 最主要的区别就是一种是把二维码图片存放在某个目录下,一种通过输出流直接返回到网页上
* 其他都是一些小区别,比如有没有imgPath或needCompress这些参数
*/
/**
* 生成二维码
* @param content 内容
* @param imgPath LOGO地址
* @param destPath 存放目录
* @param needCompress 是否压缩
* @throws Exception
*/
public static void encode(String content, String imgPath, String destPath, boolean needCompress) throws Exception {
BufferedImage image = QRCodeUtil.createImage(content, imgPath, needCompress);
mkdirs(destPath);
ImageIO.write(image, FORMAT_NAME, new File(destPath));
}
public static BufferedImage encode(String content, String destPath, boolean needCompress) throws Exception {
BufferedImage image = QRCodeUtil.createImage(content, destPath, needCompress);
return image;
}
/**
*
* @param content 内容
* @param imgPath LOGO地址
* @param output 输出流
* @param needCompress 是否压缩
* @throws Exception
*/
public static void encode(String content, String imgPath, OutputStream output, boolean needCompress)
throws Exception {
BufferedImage image = QRCodeUtil.createImage(content, imgPath, needCompress);
ImageIO.write(image, FORMAT_NAME, output);
}
public static void encode(String content, OutputStream output) throws Exception {
QRCodeUtil.encode(content, null, output, false);
}
/**
* 解析二维码
* @param file 二维码图片
* @return String
* @throws Exception
*/
public static String decode(File file) throws Exception {
BufferedImage image;
image = ImageIO.read(file);
if (image == null) {
return null;
}
BufferedImageLuminanceSource source = new BufferedImageLuminanceSource(
image);
BinaryBitmap bitmap = new BinaryBitmap(new HybridBinarizer(source));
Result result;
Hashtable hints = new Hashtable();
hints.put(DecodeHintType.CHARACTER_SET, CHARSET);
result = new MultiFormatReader().decode(bitmap, hints);
String resultStr = result.getText();
return resultStr;
}
/**
* 解析二维码
* @param path 二维码图片地址
* @return String
* @throws Exception
*/
public static String decode(String path) throws Exception {
return QRCodeUtil.decode(new File(path));
}
}
3.编写控制层代码
QrCodeController.java:
写了三个方法:
1.根据text生成普通二维码;
2.根据text生成带有logo二维码;
3.生成二维码并存放到某文件夹下,还加了一个解析的方法;
package com.erweima.controller;
import com.erweima.Utils.QRCodeUtil;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import javax.servlet.ServletOutputStream;
import javax.servlet.http.HttpServletResponse;
import java.io.File;
@Controller
public class QrCodeController {
/**
* 根据 url 生成 普通二维码
*/
@RequestMapping(value = "/createCommonQRCode")
public void createCommonQRCode(HttpServletResponse response, String url) throws Exception {
ServletOutputStream stream = null;
try {
stream = response.getOutputStream();
//使用工具类生成二维码
QRCodeUtil.encode(url, stream);
} catch (Exception e) {
e.getStackTrace();
} finally {
if (stream != null) {
stream.flush();
stream.close();
}
}
}
/**
* 根据 url 生成 带有logo二维码
*/
@RequestMapping(value = "/createLogoQRCode")
public void createLogoQRCode(HttpServletResponse response, String url) throws Exception {
ServletOutputStream stream = null;
try {
stream = response.getOutputStream();
String logoPath = Thread.currentThread().getContextClassLoader().getResource("").getPath()
+ "templates" + File.separator + "logo.jpg";
//使用工具类生成二维码
QRCodeUtil.encode(url, logoPath, stream, true);
} catch (Exception e) {
e.getStackTrace();
} finally {
if (stream != null) {
stream.flush();
stream.close();
}
}
}
}
4.运行并查看效果
本项目提供了三个接口,启动项目,进行演示;
1.生成普通二维码,内容为“I LOVE YOU !”
浏览器打开 http://localhost:8080/createCommonQRCode?text=I LOVE YOU ! ,生成的二维码截图如下:
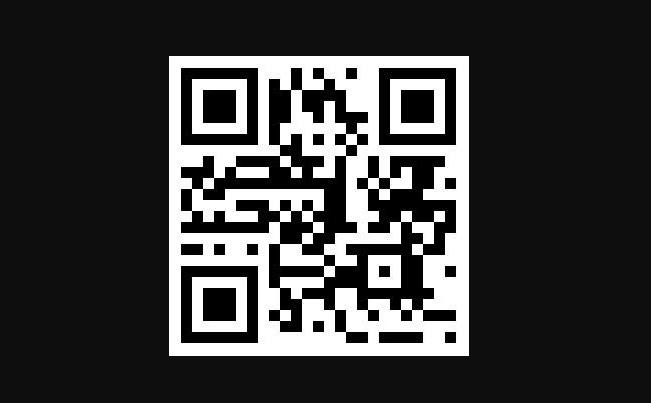
2.生成带logo的二维码,内容为“https://blog.csdn.net/Ace_2”
浏览器打开 http://localhost:8080/createLogoQRCode?text=https://blog.csdn.net/Ace_2 ,生成的二维码截图如下:
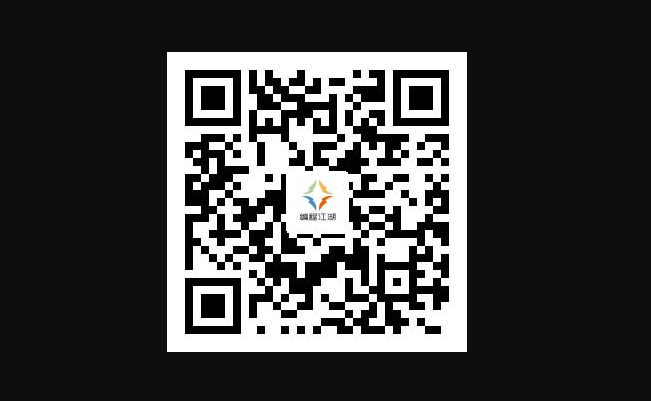
3.生成存放到硬盘的二维码图片,并用代码解析。内容为“5L中单,不给就送 !”
浏览器打开 http://localhost:8080/createSaveQRCode?text=https://blog.csdn.net/Ace_2 ,
生成好了:

打开:
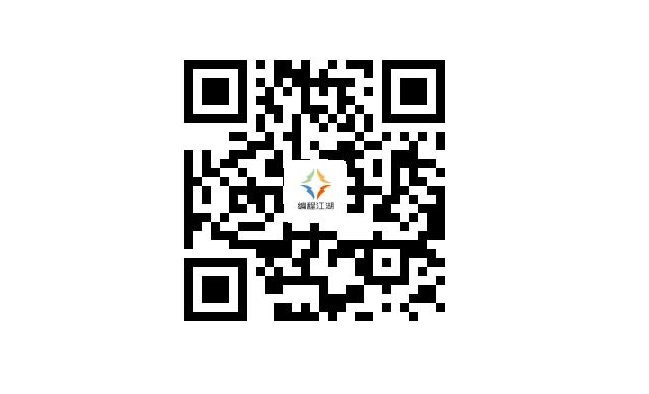
解析结果打印在控制台:

扫描结果就自己验证了吧,有问题欢迎交流哦!
----------------------------------------
欢迎关注公众号“编程江湖”,可以领取Java、Python、微信小程序等学习资料和项目源码,还能查看技术文章并和大家交流。
(完)
作者:编程江湖